It would be possible to have the slots to which the resized and moved signals are connected check the new position or size of the circle and respond accordingly, but it's more convenient and requires less knowledge of circles by the slot functions if the signal that is sent can include that information. In this tutorial we will learn How to use signal and slots in qt. File-New File or Project Applications-Qt Gui Application-Choose We keep the class as MainWindow as given by default.
last modified July 16, 2020
In this part of the PyQt5 tutorial we learn some basic functionality. The examples show atooltip and an icon, close a window, show a message box and centera window on the desktop.
PyQt5 simple example
This is a simple example showing a small window. Yet we can do a lotwith this window. We can resize it, maximise it or minimise it. This requiresa lot of coding. Someone already coded this functionality. Because it is repeatedin most applications, there is no need to code it over again. PyQt5 is a highlevel toolkit. If we would code in a lower level toolkit, the following codeexample could easily have hundreds of lines.
The above code example shows a small window on the screen.
Here we provide the necessary imports. The basic widgets arelocated in PyQt5.QtWidgets
module.
Every PyQt5 application must create an application object.The sys.argv
parameter is a listof arguments from a command line. Python scripts can be run fromthe shell. It is a way how we can control the startup of our scripts.
The QWidget
widget is the base class of all userinterface objects in PyQt5. We provide the default constructor for QWidget
.The default constructor has no parent. A widget with no parent is called a window.
The resize()
method resizes the widget.It is 250px wide and 150px high.
The move()
method moves the widget to a positionon the screen at x=300, y=300 coordinates.
We set the title of the window with setWindowTitle()
. The title isshown in the titlebar.
The show()
method displays the widget on the screen. A widget isfirst created in memory and later shown on the screen.
Finally, we enter the mainloop of the application. The event handling startsfrom this point. The mainloop receives events from the window system anddispatches them to the application widgets. The mainloop ends if we call theexit()
method or the main widget is destroyed. The sys.exit()
methodensures a clean exit. The environment will be informed how the applicationended.
The exec_()
method has an underscore. It is becausethe exec
is a Python keyword. And thus, exec_()
was used instead.
An application icon
The application icon is a small image which is usually displayedin the top left corner of the titlebar. In the following example we willshow how we do it in PyQt5. We will also introduce some new methods.
Some environments do not display icons in the titlebars. We need to enable them.See my answeron Stackoverflow for a solution, if you are seeing no icons.

The previous example was coded in a procedural style. Python programming languagesupports both procedural and object oriented programming styles. Programming inPyQt5 means programming in OOP.
Three important things in object oriented programming are classes,data, and methods. Here we create a new class called Example
.The Example
class inherits from the QWidget
class.This means that we call two constructors: the first one for the Example
class and the second one for the inherited class. The super()
method returns the parent object of the Example
class and we call its constructor.The __init__()
method is a constructor method in Python language.
The creation of the GUI is delegated to the initUI()
method.
All three methods have been inherited from the QWidget
class.The setGeometry()
does two things: it locates the window on the screen and setsit size. The first two parameters are the x and ypositions of the window. The third is the width and the fourth is the height of thewindow. In fact, it combines the resize()
and move()
methodsin one method. The last method sets the application icon. To do this, we have created aQIcon
object. The QIcon
receives the path to our icon to be displayed.
The application and example objects are created. The main loop is started.
Showing a tooltip in PyQt5
We can provide a balloon help for any of our widgets.
In this example, we show a tooltip for two PyQt5 widgets.
This static method sets a font used to render tooltips.We use a 10pt SansSerif font.
To create a tooltip, we call the setTooltip()
method. We can userich text formatting.
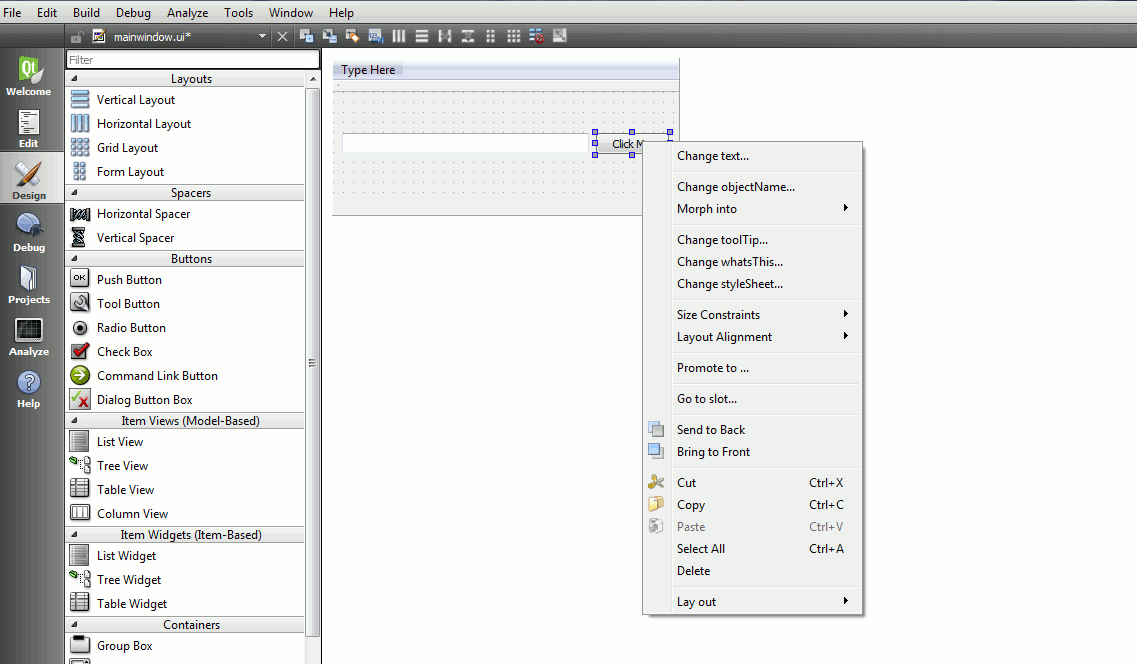
We create a push button widget and set a tooltip for it.
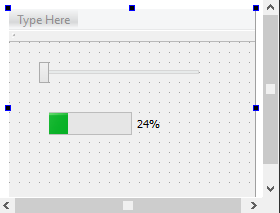
The button is being resized and moved on the window. The sizeHint()
method gives a recommended size for the button.
Closing a window
The obvious way to close a window is to click on the x mark on the titlebar.In the next example, we show how we can programatically close our window.We will briefly touch signals and slots.
The following is the constructor of a QPushButton
widget that we usein our example.
The text
parameter is a text that will be displayed on the button.The parent
is a widget on which we place our button.In our case it will be a QWidget
. Widgets of an application form a hierarchy.In this hierarchy, most widgets have their parents. Widgets without parents are toplevel windows.
In this example, we create a quit button. Upon clicking onthe button, the application terminates.
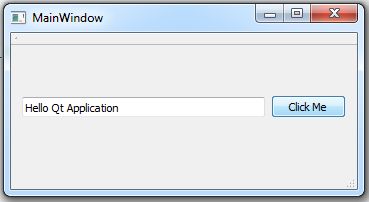
We create a push button. The button is an instance of the QPushButton
class. The first parameter of the constructor is the label of the button.The second parameter is the parent widget. The parent widget is theExample
widget, which is a QWidget
by inheritance.
The event processing system in PyQt5 is built with the signal & slotmechanism. If we click on the button, the signal clicked
isemitted. The slot can be a Qt slot or any Python callable.
QCoreApplication
, which is retrieved withQApplication.instance()
, contains the main event loop—it processesand dispatches all events. The clicked signal is connected to thequit()
method which terminates the application. The communicationis done between two objects: the sender and the receiver. The sender is the pushbutton, the receiver is the application object.
PyQt5 message box
By default, if we click on the x button on the titlebar,the QWidget
is closed. Sometimes we want to modifythis default behaviour. For example, if we have a file opened in an editorto which we did some changes. We show a message box to confirm the action.
If we close a QWidget
, the QCloseEvent
is generated. To modify the widget behaviour we need to reimplementthe closeEvent()
event handler.
We show a message box with two buttons: Yes and No. The first string appearson the titlebar. The second string is the message text displayed by the dialog.The third argument specifies the combination of buttons appearing in the dialog.The last parameter is the default button. It is the button which has initiallythe keyboard focus. The return value is stored in the reply
variable.
Here we test the return value. If we click the Yes button, we acceptthe event which leads to the closure of the widget and to thetermination of the application. Otherwise we ignore the close event.
Centering window on the screen
The following script shows how we can center a window on the desktop screen.
The QDesktopWidget
class provides information aboutthe user's desktop, including the screen size.
The code that will center the window is placed in the customcenter()
method.
We get a rectangle specifying the geometry of the main window.This includes any window frame.
We figure out the screen resolution of our monitor.And from this resolution, we get the center point.
Our rectangle has already its width and height. Now we set the centerof the rectangle to the center of the screen.The rectangle's size is unchanged.
We move the top-left point of the application window to thetop-left point of the qr rectangle, thus centering thewindow on our screen.
In this part of the PyQt5 tutorial, we have created simple code examplesin PyQt5.
In this tutorial we will learn How to use signal and slots in qt.
File->New File or Project…
Applications->Qt Gui Application->Choose…
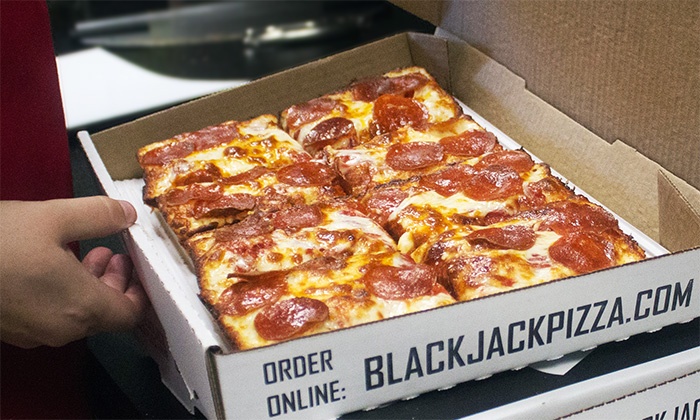
The previous example was coded in a procedural style. Python programming languagesupports both procedural and object oriented programming styles. Programming inPyQt5 means programming in OOP.
Three important things in object oriented programming are classes,data, and methods. Here we create a new class called Example
.The Example
class inherits from the QWidget
class.This means that we call two constructors: the first one for the Example
class and the second one for the inherited class. The super()
method returns the parent object of the Example
class and we call its constructor.The __init__()
method is a constructor method in Python language.
The creation of the GUI is delegated to the initUI()
method.
All three methods have been inherited from the QWidget
class.The setGeometry()
does two things: it locates the window on the screen and setsit size. The first two parameters are the x and ypositions of the window. The third is the width and the fourth is the height of thewindow. In fact, it combines the resize()
and move()
methodsin one method. The last method sets the application icon. To do this, we have created aQIcon
object. The QIcon
receives the path to our icon to be displayed.
The application and example objects are created. The main loop is started.
Showing a tooltip in PyQt5
We can provide a balloon help for any of our widgets.
In this example, we show a tooltip for two PyQt5 widgets.
This static method sets a font used to render tooltips.We use a 10pt SansSerif font.
To create a tooltip, we call the setTooltip()
method. We can userich text formatting.
We create a push button widget and set a tooltip for it.
The button is being resized and moved on the window. The sizeHint()
method gives a recommended size for the button.
Closing a window
The obvious way to close a window is to click on the x mark on the titlebar.In the next example, we show how we can programatically close our window.We will briefly touch signals and slots.
The following is the constructor of a QPushButton
widget that we usein our example.
The text
parameter is a text that will be displayed on the button.The parent
is a widget on which we place our button.In our case it will be a QWidget
. Widgets of an application form a hierarchy.In this hierarchy, most widgets have their parents. Widgets without parents are toplevel windows.
In this example, we create a quit button. Upon clicking onthe button, the application terminates.
We create a push button. The button is an instance of the QPushButton
class. The first parameter of the constructor is the label of the button.The second parameter is the parent widget. The parent widget is theExample
widget, which is a QWidget
by inheritance.
The event processing system in PyQt5 is built with the signal & slotmechanism. If we click on the button, the signal clicked
isemitted. The slot can be a Qt slot or any Python callable.
QCoreApplication
, which is retrieved withQApplication.instance()
, contains the main event loop—it processesand dispatches all events. The clicked signal is connected to thequit()
method which terminates the application. The communicationis done between two objects: the sender and the receiver. The sender is the pushbutton, the receiver is the application object.
PyQt5 message box
By default, if we click on the x button on the titlebar,the QWidget
is closed. Sometimes we want to modifythis default behaviour. For example, if we have a file opened in an editorto which we did some changes. We show a message box to confirm the action.
If we close a QWidget
, the QCloseEvent
is generated. To modify the widget behaviour we need to reimplementthe closeEvent()
event handler.
We show a message box with two buttons: Yes and No. The first string appearson the titlebar. The second string is the message text displayed by the dialog.The third argument specifies the combination of buttons appearing in the dialog.The last parameter is the default button. It is the button which has initiallythe keyboard focus. The return value is stored in the reply
variable.
Here we test the return value. If we click the Yes button, we acceptthe event which leads to the closure of the widget and to thetermination of the application. Otherwise we ignore the close event.
Centering window on the screen
The following script shows how we can center a window on the desktop screen.
The QDesktopWidget
class provides information aboutthe user's desktop, including the screen size.
The code that will center the window is placed in the customcenter()
method.
We get a rectangle specifying the geometry of the main window.This includes any window frame.
We figure out the screen resolution of our monitor.And from this resolution, we get the center point.
Our rectangle has already its width and height. Now we set the centerof the rectangle to the center of the screen.The rectangle's size is unchanged.
We move the top-left point of the application window to thetop-left point of the qr rectangle, thus centering thewindow on our screen.
In this part of the PyQt5 tutorial, we have created simple code examplesin PyQt5.
In this tutorial we will learn How to use signal and slots in qt.
File->New File or Project…
Applications->Qt Gui Application->Choose…
Call (970) 669-1717 or email Blackjack Pizza in Loveland and let us know how we can help! We always love hearing what we can do for our customers. Handcrafted, crunchy crust, smothered in cheese. Order Blackjack Pizza online for delivery or pick-up. See our coupons page for current deals and discounts. Pizza in Dallas, TX. 104th AveThornton, CO 80233. ONLINE ORDERING. This field is for validation purposes and should be left unchanged. Tasty Deals Just For You.
We keep the class as MainWindow as given by default.
SignalsAndSlots.pro
2 4 6 8 10 12 14 16 18 | #include 'ui_mainwindow.h' MainWindow::MainWindow(QWidget*parent): ui(newUi::MainWindow) ui->setupUi(this); connect(ui->horizontalSlider,SIGNAL(valueChanged(int)), disconnect(ui->horizontalSlider,SIGNAL(valueChanged(int)), } MainWindow::~MainWindow() delete ui; |
main.cpp
2 4 6 8 10 | #include intmain(intargc,char*argv[]) QApplicationa(argc,argv); w.show(); returna.exec(); |